Names
This lab is about names: how naming things can be a powerful tool for thinking, and how to assign names to things in Python. One part philosophy, one part programming! There are a few things to do before you start. This setup will be the same for every lab from now on.
- ๐ป
Run
mwc update
. - ๐ป
Move into the lab directory:
cd Desktop/making_with_code/mwc1/unit1/lab_names
. - ๐ป
Run
poetry shell
to enter the Python environment for this lab.
Variables: Naming values
A value is anything that is what it is, instead of referring to something else.
In the turtle lab, you worked with integer
values like 1
, 100
, and 65
. Python has other types of values too, things like
3.1416
, "hello"
, True
, and False
.
Let's play with some values, using Python's interactive mode.
๐ป
Run python
. You will see >>>
, Python's interactive
prompt. Try out some common mathematical operators, which combine values to
create new values:
>>> 10 + 10
20
>>> 100 - 99
1
>>> 6 * 4
24
>>> 72 / 9
8
A name is different from a value, because it refers to something else. For example, your name refers to you. But you are not the same as your name. You could change your name, and you would still be the same person. You might even be called by different names in different contexts.
A variable is a name that refers to a value. In Python, we
assign a value to a variable by writing name = value
. Take note:
this use of =
is very different from in math class! In math class,
=
is a statement expressing that two things are equal, such as
2 * 5 = 10
. Here, we are not expressing that two things are equal.
We are assigning the value on the right to the name on the left.
Once you assign a value to a variable, you can use the variable's name
instead of its value.
๐ป Try doing some math with variables instead of values. Here, we calculate the area of a circle.
>>> pi = 3.141592653
>>> radius = 10
>>> circle_area = pi * radius * radius
>>> circle_area
314.1592653
๐ป
Exit interactive mode by running exit()
(Control + D
works too). Open greetings.py
by running
code greetings.py
. It's a simple program:
my_name ="Chris"
greeting = "Hello, " + my_name
print(greeting)
๐ป
What do you think this program will print?
Run python greetings.py
.
๐ป
However, your name probably isn't Chris. Change
greetings.py
so it says hello to you, save it, and run it again.
๐ป
Now let's make greetings.py
more flexible.
Change the first line of the program to:
my_name = input("What is your name? ")
Run the program again--now the program uses the
input
function to get a value from the user, and assigns whatever
value the user typed in to my_name
. By using the variable's name,
the program can interact with an unknown value
(the program can't know what the user will enter ahaed of time).
๐ป
circle_area.py
is a program which calculates
the area of a circle, but it isn't finished. Finish the program, so
that it prints out the area of the circle. Once the program is
finished, it should work like this:
$ python circle_area.py
This program will calculate the area of a circle.
What is the circle's radius? 5
78.539816325
Functions: Naming code blocks
A code block is one or more lines of code. Here's a code block that draws a square:
1 from turtle import *
2
3 forward(100)
4 right(90)
5 forward(100)
6 right(90)
7 forward(100)
8 right(90)
9 forward(100)
10 right(90)
You could name this block of code by defining a function:
1 from turtle import *
2
3 def square():
4 forward(100)
5 right(90)
6 forward(100)
7 right(90)
8 forward(100)
9 right(90)
10 forward(100)
11 right(90)
12
13 square()
14 forward(100)
15 square()
16 forward(100)
17 square()
Take note of line 3: to define a function, we use the def
keyword,
then the function's name, then ()
, then :
. The function's
code block is indented on the following lines.
Defining a function assigns a name to a block of code, but it doesn't
run the block of code. A function's code block doesn't run until the
function is called. In the example above, square
is called three times,
on lines 13, 15, and 17. One reason for defining functions is to manage
complexity. Once you have defined square
(and tested it to make sure it's correct),
you don't need to worry anymore about how it works. You can just
call square()
, and spend your attention on what you're trying to do with squares.
Functions with arguments
You might have noticed that square
only draws one size of square, even though
the code would be almost exactly the same for a square of a different size.
To make square
adjustable, we can change its definition so it takes an argument.
1 from turtle import *
2
3 def square(side_length):
4 forward(side_length)
5 right(90)
6 forward(side_length)
7 right(90)
8 forward(side_length)
9 right(90)
10 forward(side_length)
11 right(90)
12
13 square(100)
14 square(80)
15 square(60)
An argument is a temporary variable which gets defined when a function is
called. Instead of ()
on line 3, we now have (side_length)
. Because square
now has one argument, it must be called with one value, as you can see on lines
13, 14, and 15. On line 13, square(100)
calls square
,
temporarily assigning the variable side_length = 100
. When square(80)
is called
on the next line, square
is called again, but this time, side_length = 80
.
side_length
does not exist as a variable outside of square
.
๐ป
Run code shapes.py
to open shapes.py
.
Two functions are defined:
triangle
(which takes one argument), and rectangle
(which takes two arguments).
Neither function is finished--each code block is just pass
, which means "do nothing."
Replace pass
with a code block which draws the correct shape for each function.
Note that shapes.py
defines triangle
and rectangle
, but does not call them. In fact,
if you run python shapes.py
, nothing happens. Instead, test your functions by running:
$ python test_shapes.py
Once this looks reasonable, run draw_with_shapes.py
, which calls triangle
and rectangle
repeatedly to produce a nice effect.
$ python draw_with_shapes.py
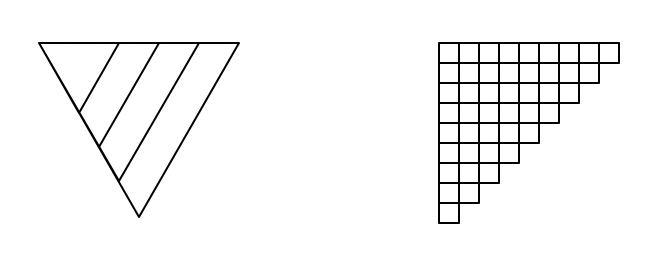