Iteration
Lab setup
First, make sure you have completed the initial setup.
If you are part of a course
-
Open Terminal. Run the update command to make sure you have the latest code.
$ mwc update
-
Move to this lab's directory and enter the lab's shell environment.
$ cd ~/Desktop/making_with_code/mwc1/unit1/lab_iteration $ poetry shell
If you are working on your own
-
Move to your MWC directory.
$ cd ~/Desktop/making_with_code
-
Get a copy of this lab's materials.
$ git clone https://git.makingwithcode.org/mwc/lab_iteration.git
-
Move into the lab directory, install the dependencies, and enter the lab's shell environment.
$ cd lab_iteration $ poetry install $ poetry shell
Don't forget to get setup before you start:
- ๐ป
Run
mwc update
. - ๐ป
Move into the lab directory:
cd Desktop/making_with_code/mwc1/unit1/lab_iteration
. - ๐ป
Run
poetry shell
to enter the Python environment for this lab.
In the Names Lab, you learned how
to write functions with arguments, such as square
:
1 from turtle import *
2
3 def square(side_length):
4 forward(side_length)
5 right(90)
6 forward(side_length)
7 right(90)
8 forward(side_length)
9 right(90)
10 forward(side_length)
11 right(90)
This is a powerful function--instead of repeating the code block every time you
want to draw a square, you can just call square(50)
, square(100)
, or whatever
size square you want.
But what if you want to draw a lot of squares? In this lab you will learn about iteration, the process of doing something multiple times.
Lists
A list is a data structure which holds any number of values, separated by commas and
surrounded by square brackets. For example, []
, [50]
, and [100, 200, 300, 400]
are all lists of numbers.
Once you create a list, you can iterate through it using a "for-loop".
(Iteration is the process of going through a collection of things one at a time.)
Line 13 goes through sizes
, one number at a time. Each number is temporarily assigned
to the variable size
, and then the code block runs.
12 sizes = [20, 40, 60, 80, 100]
13 for size in sizes:
14 square(size)
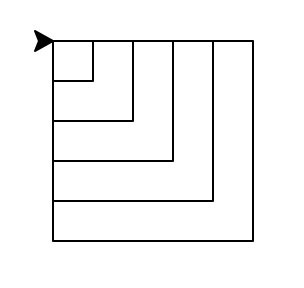
Sometimes we just want to repeat the code block, and we don't even use the temporarily-assigned
variable. The example below doesn't use the temporarily-assigned variable size
, so the values
of the numbers in the list don't matter. All that matters is how many numbers there are in the list.
15 for size in sizes:
16 square(20)
17 penup()
18 forward(40)
19 pendown()
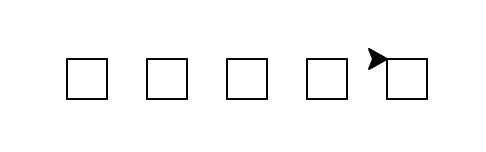
Ranges
Lists are great, but what if you want to draw a lot of squares? You don't
necessarily want to type out a 100-item list just to iterate over it.
Python has a built-in function called range
which automatically creates a
list-like object. range
can be called in a few different ways.
๐ป
Run python
to open Python's interactive mode. Enter the
following commands to try out using ranges.
range(max)
generates the numbers from 0
up to max
(not including max
).
>>> for number in range(5):
... print(number)
...
0
1
2
3
4
range(min, max)
generates the numbers from min
up to max
(not including max
)
>>> for number in range(5, 10):
... print(number)
...
5
6
7
8
9
range(min, max, stride)
generates the numbers from min
up to max
, counting by stride
>>> for number in range(0, 100, 20):
... print(number)
...
0
20
40
60
80
Draw with ranges
drawtiles.py
provides an example of what you can do with ranges. This program
takes a function to draw a single tile, draw_tile
from tile.py
, and draws
it over and over in a grid.
๐ป
Run python drawtiles.py --help
to find out how to use
this program.
(lab-iteration-py3.11) lab_iteration % python drawtiles.py --help
usage: python drawtiles.py [-h] [--fast] width height size
Draws a grid of tiles.
positional arguments:
width How many tiles across the grid should be
height How many tiles high the grid should be
size Side length of each tile
options:
-h, --help show this help message and exit
--fast Skip turtle animation and show the result
The first line of the help message shows how to run the program.
You need to provide three arguments: width, height, and size, and can optionally
also add the --fast
flag. The rest of the help message explains what each argument
does.
๐ป
Run python drawtiles.py 5 3 40
. If you're impatient, add --fast
.
Try running drawtiles.py
with different arguments. Then open tile.py
and try
changing the draw_tile()
function. Create your own tile pattern.